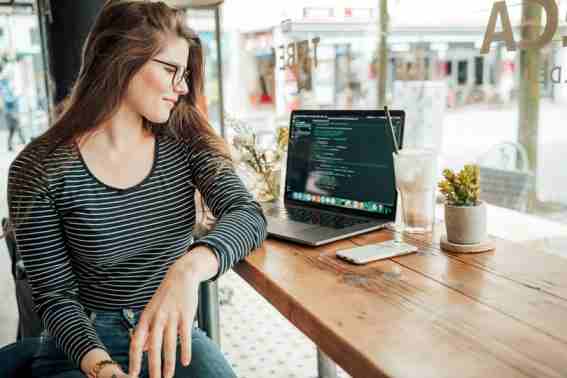
Table of Contents
Introduction
In modern web development, efficiency and simplicity often go hand-in-hand when managing and processing data. Laravel, one of the leading PHP frameworks, offers a powerful feature called ‘pipeline’ for streamlining complex data operations. This tool enables developers to pass data through a sequence of classes and manipulate it elegantly and readably. Laravel pipelines are particularly useful for applying a series of filters or performing actions that need to be decoupled from the main logic of an application. In this article, we’ll explore how Laravel pipelines work and how you can implement them to improve your data workflow.
Setting Up Your First Laravel Pipeline
Embarking on the creation of your first Laravel pipeline is a straightforward process. It begins with defining the series of pipes, which can be done within a service provider or directly within the method that requires the pipeline. You’ll then instantiate the ‘Pipeline’ class, supplying it with the list of pipes you’ve created.
The Laravel ‘Pipeline’ class provides several methods allowing you to custom-tailor the passage of data. The ‘send’ method is used to set the initial piece of data you want to process, and the ‘through’ method allows you to specify the array of pipes the data should pass through. The ‘then’ method is used to finalize the pipeline, return the modified data, or progress to further actions.
You can use different types of callable formats when setting up your pipes. This can range from simple Closure functions to more complex invokable class structures. Regardless of the method, the premise remains the same – each pipe receives the data and returns it modified or interacts with it.
Getting this right frees you up to reuse your pipeline across different parts of your application. Each part of the process can then focus on its task without worrying about the broader context, leading to more modular and less interdependent code.
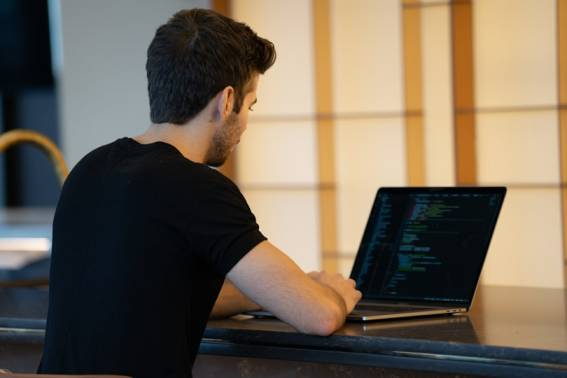
Applying Filters to Data in Laravel Using Pipeline
One of the most common use cases for Laravel pipelines is to apply a set of filters to a dataset, especially when building complex search or report generation features. For example, imagine you have an e-commerce application where you want to filter products based on category, price, and availability.
Each filter would be a separate pipe within your pipeline. As your product collection flows through the pipeline, it gets filtered down until you’re left with only the items that match all the given criteria. This is both performant and highly expressive, as each filter defines a single facet of your filtering requirements.
Returning the data correctly at each stage ensures the pipeline flows smoothly. One standard convention is to pass the data along with a ‘next’ closure that pipes can call to pass the data down the line. If a pipe doesn’t need to process the data, it simply calls ‘next’ without any alterations.
Incorporating a Laravel pipeline into your application benefits it greatly by allowing for flexible and dynamic data manipulation. This enhances your application’s overall scalability and maintainability, easily accommodating future complexity.
Advanced Techniques in Data Processing with Laravel Pipeline
Laravel pipelines can be extended with advanced techniques for sophisticated data processing needs. This includes conditionally adding pipes based on the context of your application or making dynamic changes to the pipeline based on the incoming data.
Another powerful capability is the use of pipelines within pipelines. For exceptionally complex scenarios, smaller pipelines can be nested within larger ones to group logical operations. This hierarchical structuring neatly encapsulates related processes, making the overarching pipeline more understandable and less cluttered.
Pipeline techniques also allow for early termination, where a pipe can stop the execution and return a response immediately. This is particularly useful in cases such as authorization, where you might want to cease operation if a user doesn’t have the necessary permissions to continue.
Lastly, exception handling can be built within pipes to manage errors gracefully without breaking the pipeline’s flow. Careful planning of such mechanisms ensures robustness in your application by providing fallbacks or alternative paths if something goes wrong during the processing stages.
Altogether, Laravel pipelines offer a powerful and modular approach to managing data processing and filtering, enhancing performance and readability. By leveraging these pipelines, developers can build more scalable and maintainable applications, quickly adapting to evolving requirements and complex data operations.